It’s fairly easy to get started using iOS Native components in React Native. At the time of writing, this is the example given in the React Native docs.
It’s straightforward – you subclass RCTViewManager
and implement a view
method that returns a UIView
. Obviously you’ll soon want to start creating more custom views. Like this UITextView
, for example:
…then we can import our native view in React Native…
And our result will look something like this:
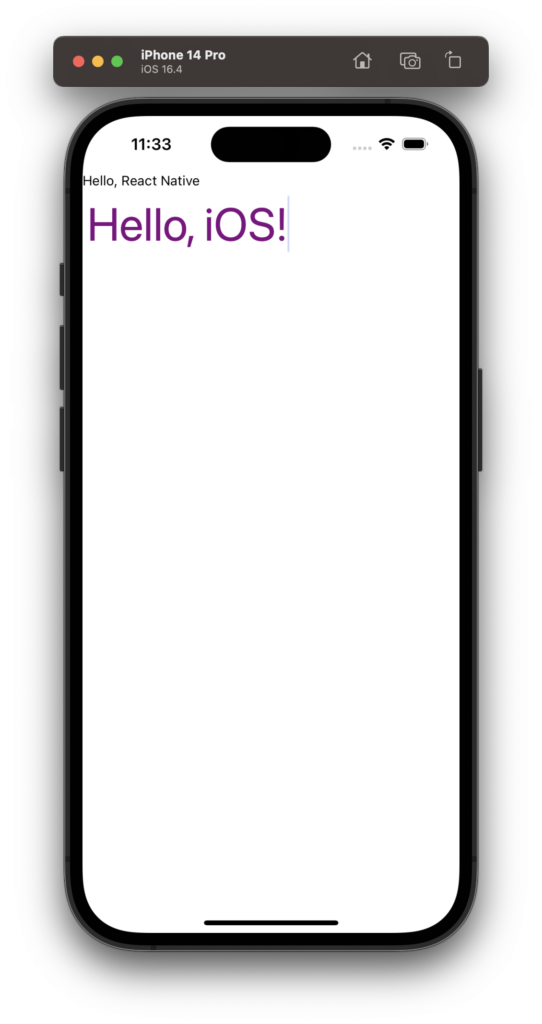
Nice! This is a good start towards learning to build custom components, but if you’re like me, you like to avoid Objective-C as much as possible, and would prefer to use Swift when you write iOS native code. Here’s how we’d do that.
First, let’s create a swift file. I’ll call it TextViewProvider
. When you create this file in Xcode, you should be asked whether you’d like to create a bridging header (if your project doesn’t already have one). Answer yes to this; the bridging header is what will make your Swift code available to your Objective-C code.
Our new file will look this this, which creates the same type of UITextView
as our original code, but in Swift.
…then, in our main RCTViewManager
class, we can make a couple of tweaks to get our view from the new swift class:
Notice how we’ve imported SwiftViewSchool-Swift.h
in our file. This is a reference our bridging header, and it’s how our Objective-C code is able to access our Swift class. The naming convention for the import is {YourProjectName}-Swift.h
. You can see the header name under Build Settings > Swift Compiler – General.
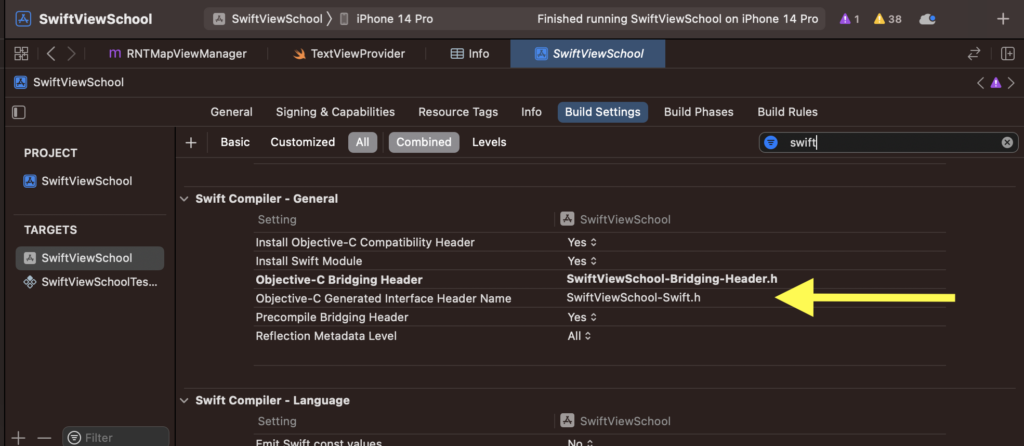
Now, when we rebuild our app, we should the text view we created in Swift.
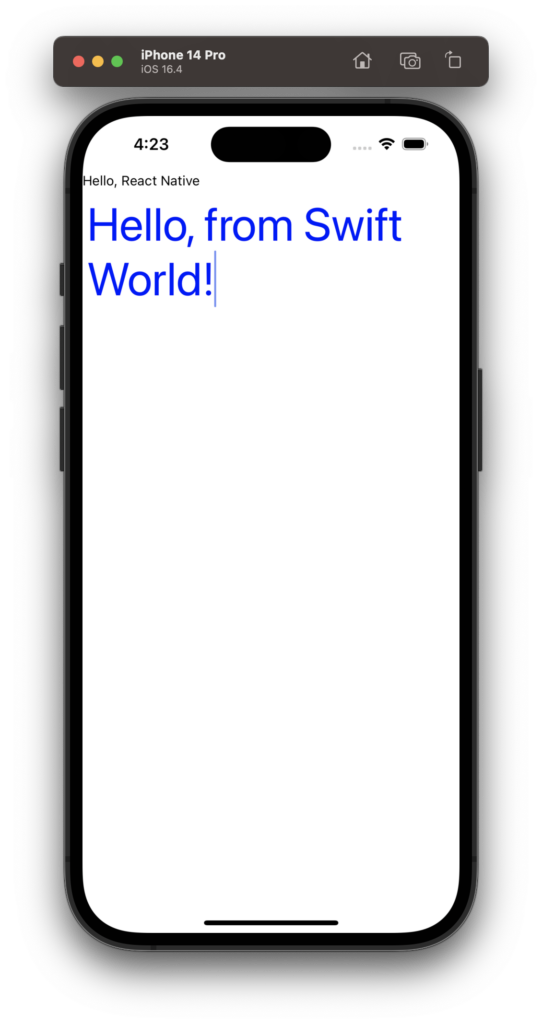
Another approach would be to subclass UITextView
with our Swift class: